
Imagine this scenario. You go to a the grocery store to purchase a few items. You decide to grab some beers, because… beer! You get to the front of the line and the cashier says: “I’m sorry, alcoholic beverage purchases need to go to cashier #5”. Hmmm. Weird, but ok. You go to cashier #5 and wait in line again. When you get to the cashier s/he asks you nicely to show your license. You do, and the cashier replies: “Perfect. You are cleared to purchase this item, now please go to the back of the line to finalize your purchase.” — WHAT!?
This is the default state of user logins in WordPress.
By default, if you want your registered users to login to your site, you have to point them to /wp-admin
.
Then, when they login there, they get directed to /wp-admin/profile.php
Most end-users are now completely lost. They don’t know why or how they got where they are, but that profile form with the weird colorbars at the top is not going to get them to the premium content or plugin that they were trying to purchase. Most e-commerce or membership plugins handle user logins fairly robustly. But often, even the default for them is to simply point the user to the homepage rather than the backend profile.
Besides all of that, sometimes you just need to route different users for different reasons and honestly, why does it have to be so hard? It doesn’t.
This tutorial will show you how to create conditional custom login redirects in WordPress for any user from any page, to any page you like. And all of that in 16 lines of code.
Creating Conditional Custom Login Redirects in WordPress
TL;DR
If you’re a WordPress developer and hate to read, here you go. Or you can check out this Gist with full inline documentation.
function wip_login_redirect( $url, $request, $user ){ $slug = $_SERVER["REQUEST_URI"]; $islogin = strpos($slug, 'log-in'); if( $user && is_object( $user ) && is_a( $user, 'WP_User' ) ) { if(($user->has_cap('edit_users')) && ($islogin==true)) { $url = admin_url('index.php'); } elseif ((!$user->has_cap('edit_users')) && ($islogin==true)) { $url = home_url(); } else { $url = $slug; } } return $url; } add_filter('login_redirect', 'wip_login_redirect', 10, 3 );
Give Your Users a Real “Logged In Experience”
The function above simply extends a filter called “login_redirect” which WordPress leverages for the $redirect_to variable. That’s a really powerful variable/filter that really needs better documentation on the Codex. But I always find it better to understand the value of a function by understanding what it does for your users. In this case, this function helps us give our users what I call a real “Logged In Experience”. Meaning, no matter where they are coming from, we’ll help get them where they want to go.
There are a lot of ways to think about the whole “Logged In Experience”, and many other tools that should be considered as well. But this one function helped me think through that for WordImpress. So here’s my suggestions for you as you develop the “Logged In Experience” for your users.
Make your “Door” Welcoming and Inviting
Everyone loves to pour all kinds of love onto their homepage, and for good reason. But as soon as you start allowing registrations, you need to realize that you now have a second “door”. Your login page is a portal for your users who are invested in your business. They’ve already trusted you with their contact information, and now they’ve come back and want to have a real “logged in” experience. If you don’t give this any thought, then these highly prized users have to go to a bland wp-login.php
page, and get redirected to a backend “Profile” page that they have no idea what to do with.
Instead, create a customized login page with a custom url. Devin wrote a detailed article a while back on creating a Custom Functionality plugin and provided some code for that there. Another handy plugin for that kind of thing is “Theme My Login“. Whatever you choose, make sure it gives you the simplicity and flexibility you need to make it look great.
Now, contrary to a house, with a website there’s just no reason for the login screen to be the only “door” to the site, particularly when you want to encourage users to log in. For us, the most important place for users to login is when they are purchasing plugins or needing support. For that reason we have login forms prominently placed on those pages as well. But you can guess already that someone who logs in at a cart wants something different from those who log in at the support page. Read on about how we handle that.
Make sure the Visitor is Someone You Want in Your Store
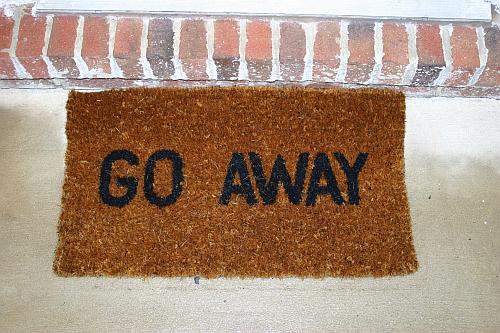
Next, while the login page should be warm and inviting, it should also be a iron gate with barbed wire and hell-hounds for anyone who shouldn’t be logging in! This post is not about security, but that is a major factor in creating your “Logged In Experience.” In our function above, we do a simple check for whether or not the person attempting to login is actually a user of the site already. WordPress itself has several other security features built into the login process, but tools like iThemes Security or leveraging two-factor authentication are also super helpful for this kind of thing.
Let the Shop Owner Get Straight to the Register
Now that the right people feel welcomed to your site, you want to find out just a little bit about them so you can direct them to where they want to go. As an Admin of A LOT of WordPress sites, I need a direct bee-line to the Dashboard. So our script above asks these questions first:
- If the person logging in is a user
- And an Admin
- And is logging in from the Login screen
- Direct them to the Dashboard
Because that’s where I want to go. Besides Devin and I, there is literally NO ONE ELSE registered in our site who needs to access the Dashboard, that’s why the function limits that specifically to Admins. The others need a different take.
If Your Guest Doesn’t Know Where to Go, Take them to the Most Important Place
But Admins are not the only users who might use the login page. We have links to the login page prominent throughout the site. Someone who logs in there might be doing that out of convenience, or they might just not know where they want to go exactly. The one thing we know for sure is they don’t need to go to the backend at all.
So next, our script asks these questions
- If the user is NOT an Admin
- And is logging in from the Login page
- Direct them to the Homepage
Our homepage is the safest place for them because it was designed as the primary portal to get anywhere else in the whole site. Plus, they will also see their login picture and name in the top right of the screen in case they are trying to get to their Profile or Purchase history.
But don’t forget, we’ve got lots of “doors” to login here. Logging in anywhere else on the site should be treated differently.
If Your Guest Knows Where to Go, Don’t Take Them Somewhere Else
Lastly, because we have login forms throughout the Support and checkout areas, we know that users who are on those pages already want to be there. They came to ask a support question or to purchase something. Why in the world should we redirect them to the Admin area or the homepage from the place they actually want to be at? We shouldn’t.
The last part of the script asks the following questions:
- If it’s a real user, whether Admin or not
- And they are NOT on the Login page
- Redirect them back to where they logged in at.
As an Admin, I don’t always log in on the login page. I often get to the site because I’m clicking on a link to a new support question. The last thing I want to do is go somewhere else to login and then go chasing that support ticket down again in order to answer it. So this is a big benefit to us Admins because we can login on the page that we need to supply an answer for. Users will appreciate it to when they land on the site to reply to their tickets and realize that they can just login there and not get pushed somewhere else either.
There you go. Now everyone gets right where they want to go without any confusion. And all of that with one function, about 20 lines long.
Thanks for posting this Matt. I haven’t had the time to look into proper redirecting logins but it’s been on my list to do for a while. I sometimes get frustrated from my own sites and others always seeing the WP brand login or improper redirects that aren’t UX friendly. Bravo for sharing!
Check out Theme My Login: https://wordpress.org/plugins/theme-my-login/ It’s useful for branding the login but sucks about redirects.
Yo Matt and Devin, wondering what you would suggest for a more complex login scenario (per user): We have a site where my clients create pages specific for their clients…those pages are locked unless a user from a group with permissions to access that page is logged in. We want my client’s clients to be redirected to their page directly after login. This obviously needs to scale as my clients will be creating groups, users and pages on the fly. I’m thinking a login condition that checks 1) they are subscriber role 2) they are a member of a group 3) that group has access to a page using template-x.php — if yes to all three send them to template-x.php Not sure how to run the check for item 3 though… Thoughts?? Currently using: Groups Plugin (https://wordpress.org/plugins/groups/)
Interesting. Off the top of my head I think this could be possible if the URL of the redirect page has a pattern that mimics the username. So you could add conditional logic based on the login-name that then points to a slug that is derivative of their login name. Put something together in a Gist with inline comments about the user slug and we can go from there.
I thought of that, but didn’t want to restrict my clients when naming groups etc. For example, they have clients with similar group names and I thought this could pose an issue. Also, when they are setting up their pages, I don’t want to have to give them naming conventions for those either. We aren’t under contract for this step of the project yet (they are adding features)…but I’ll post a gist when we get underway.
Actually… a good idea would be to attach a field to the user account called "User Slug" and that is automatically entered when they create the account based on their username. But it can be modified. Then in the redirect all you’d do is reference that metadata as the redirect url. I kinda like that idea.
I was just thinking – what if I have WP auto create a page on this action: groups_created_group. So, when a new group is created a page will be setup with my custom URL structure (based on their group name) and the correct template, page permissions etc. will be ready out of the box. In my head that seems pretty easy, ha.
how force user to login or register before checkout process
in woocommerce